Password Encryption using MD5 Hash Algorithm in C#
Simple way to hash sensitive string
Update (Sep 14, 2018): The new complete string hash algorithms in C# tutorial is available. I would suggest not to use MD5 anymore, because it’s not safe and easily collide. But I’ll leave the original tutorial here for educational purpose.
It’s really bad if someone knew your password. As a software developer, we have an ethic that we also don’t want to see other’s password. Which mean, storing password in a plain text is a nope.
So, what we need to do to store those passwords?
One simple solution is using a hash function. We change the readable password into unreadable text and no one, not even yourself, will able to decrypt that text. One of the simple method is MD5 Hash Algorithm.
How does MD5 Hash Algorithm Works? To put it simply, this algorithm change your password into 32 hexadecimal digits. It doesn’t care how long is your password is, it’ll always become 32 hexadecimal digits. For complete explanation please read this wikipedia article.
The next question is, how can we do that, specifically in .NET Framework? Well, it’s actually very easy because they already provide it for us to use. I will show you how to implement MD5 hash algorithm using .NET Cryptography Framework.
All we need to do is to create a helper class with static function.
Let’s call our class Encryptor
.
Inside this class, we’re going to create a new static function MD5Hash
.
The purpose is self-explained in the name.
But in case you’re wondering, this function will pass your sensitive string, or password, and turn it into an MD5 Hex string.
You can see following snippet for the complete implementation.
using System.Text;
using System.Security.Cryptography;
namespace CryptoLib
{
public static class Encryptor
{
public static string MD5Hash(string text)
{
MD5 md5 = new MD5CryptoServiceProvider();
//compute hash from the bytes of text
md5.ComputeHash(ASCIIEncoding.ASCII.GetBytes(text));
//get hash result after compute it
byte[] result = md5.Hash;
StringBuilder strBuilder = new StringBuilder();
for (int i = 0; i < result.Length; i++)
{
//change it into 2 hexadecimal digits
//for each byte
strBuilder.Append(result[i].ToString("x2"));
}
return strBuilder.ToString();
}
}
}
I tested that to my WPF Software, and this is the screenshot of the MD5 string result if I typed my password.
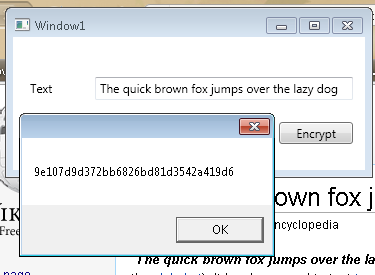
That’s it. Hopefully this simple tutorial helps you.
Thanks for reading and happy coding!
References
- Generating MD5 Hash out of C# Objects - CodeProject
Cover Photo by Jess Watters on Unsplash.